Components > Canvas
1import { Canvas } from "@/components/app-components";
🎨 Incorporate a versatile and customizable canvas component that accommodates everything from user-drawn sketches to uploaded images. Users can effortlessly select over +1000 icons and SVGs through the canvas component's built-in tabs. Perfect for developing image AI apps, design apps, and more to seamlessly integrate comprehensive visual user input into your application.
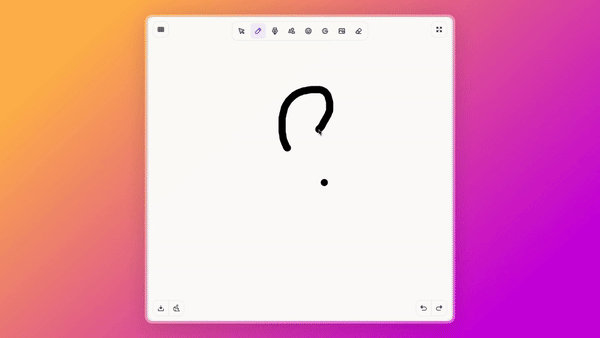
Features
- -> Drawing and editing capabilities with support for shapes, icons, brands, and custom images.
- -> Advanced features like undo/redo, clear canvas, download design, and fullscreen mode.
- -> Customizable overlay buttons to show/hide specific functionalities.
- -> Integrated with React Context for direct access to canvas features and states.
Customization
The Canvas component can be customized by setting properties in the configuration object. This allows you to control the visibility of various overlay buttons based on your application's requirements.
1
2 ...
3 canvas: {
4 hideShapes: false, // Hide the shapes button
5 hideIcons: false, // Hide the icons button
6 hideBrands: false, // Hide the brands button
7 hideUpload: false, // Hide the upload button
8 hideEraser: false, // Hide the eraser button
9 hideDownload: false, // Hide the download button
10 hideClear: false, // Hide the clear canvas button
11 hideUndoRedo: false, // Hide the undo/redo buttons
12 hideGrid: false, // Hide the grid button
13 hideFullscreen: false, // Hide the fullscreen button
14 },
15 ...
16
React Context Usage
With a simple React context CanvasContext: with many features decoupled from all the application logic, everything related to canvas can be managed just by importing the canvas context inside your application just like below:
1
2 const {
3 setMenuState, // Setter for active drawing state (select, brush, line, eraser etc.)
4 menuState, // Getter for active drawing state.
5 fabricCanvas, // The instance of the Fabric.js canvas.
6 undo, // Function to undo the last action.
7 redo, // Function to redo the last action.
8 clear, // Function to clear the canvas.
9 download, // Function to download canvas as PNG
10 brushWidth, // Getter for brush size.
11 setBrushWidth, // Setter for brush size.
12 eraserWidth, // Getter for eraser size.
13 setEraserWidth, // Setter for eraser size.
14 addSvg, // Function to add svg images to canvas.
15 addImage, // Function to add base64 encoded images to canvas.
16 addImageUrl, // Function to add remote images to canvas.
17 fullScreen, // Getter for fullscreen state.
18 setFullScreen, // Setter for fullscreen state.
19 addGridBackground, // Setter for grid state.
20 removeGridBackground, // Setter for grid state.
21 gridBackgroundActive, // Getter for grid state.
22 activeSelection, // Getter for active canvas selection.
23 setActiveSelection, // Setter for active canvas selection.
24 } = useContext(CanvasContext);
25
Usage
1
2 const ResizedCanvas = () => {
3 const { fullScreen, fabricCanvas } = useContext(CanvasContext);
4 useEffect(() => {
5 const handleResize = () => {
6 const containerWidth = 0; // calculate using document.body.clientWidth based on your needs
7 const containerHeight = 0; // calculate using document.body.clientHeight based on your needs
8
9 // To preserve square shape of the canvas
10 const min = Math.min(containerWidth, containerHeight);
11
12 const scale = min / fabricCanvas.current.getWidth();
13 if (scale === 1) return;
14 const zoom = fabricCanvas.current.getZoom() * scale;
15 fabricCanvas.current.setDimensions({
16 width: min,
17 height: min,
18 });
19 fabricCanvas.current.setViewportTransform([zoom, 0, 0, zoom, 0, 0]);
20 };
21 handleResize();
22 window.addEventListener("resize", handleResize);
23
24 return () => {
25 window.removeEventListener("resize", handleResize);
26 };
27 }, [fullScreen]);
28 return <Canvas />;
29 };
30
31 export const CanvasUsage = () => {
32 const canvasContext = useCanvasProvider();
33
34 return (
35 <CanvasContext.Provider value={canvasContext}>
36 <ResizedCanvas />
37 </CanvasContext.Provider>
38 );
39 };
40