ESC
Components > Prompt Input
page.tsx
1import { PromptInput } from "@/components/app-components";
A fully fledged and customizable prompt input component that can be used to take user input, change strength of the various sections of the prompt and trigger generating images based on the prompt.
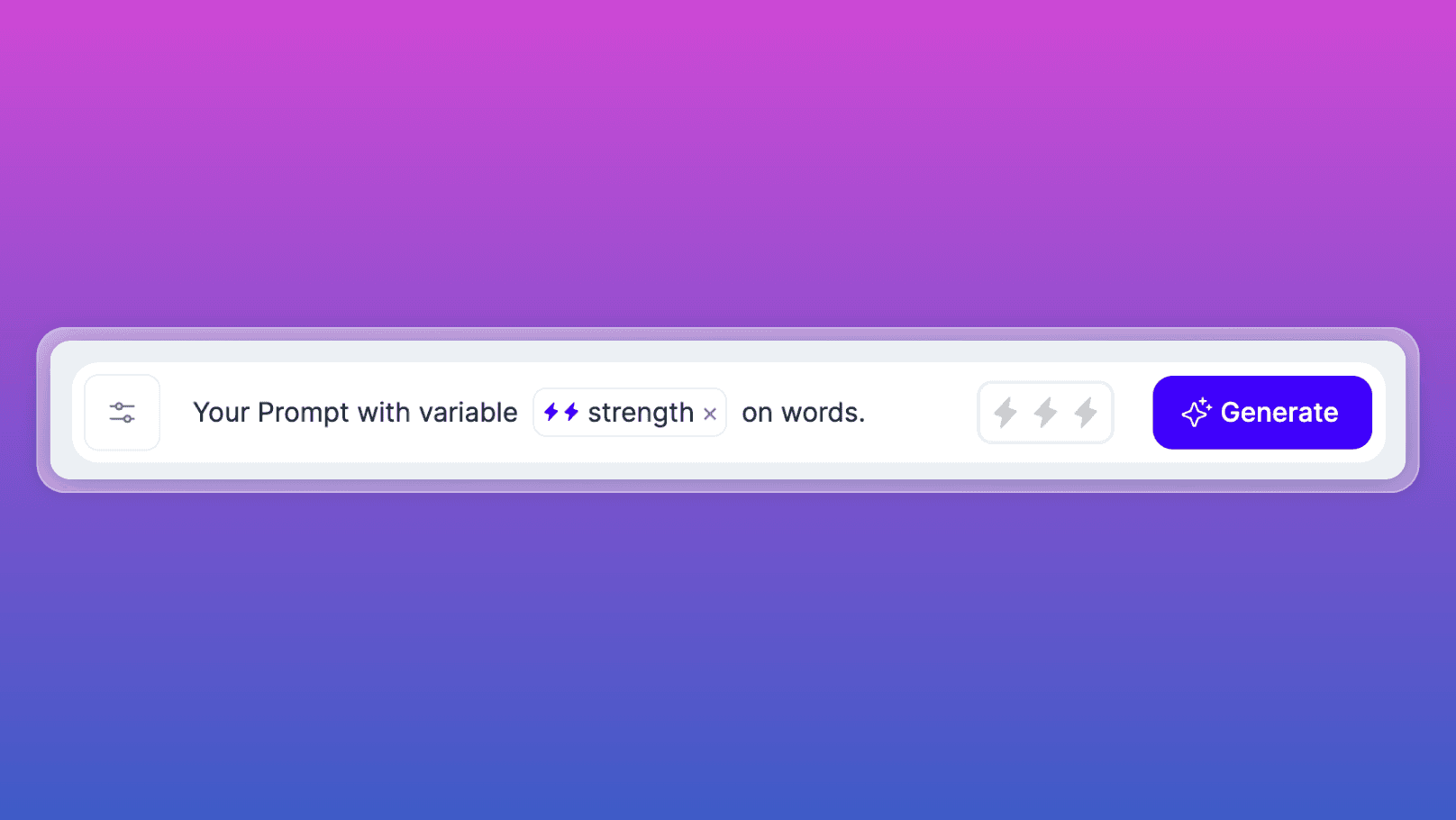
Also supports negative prompts 🤩 Perfect for apps that generate images based on user prompts!
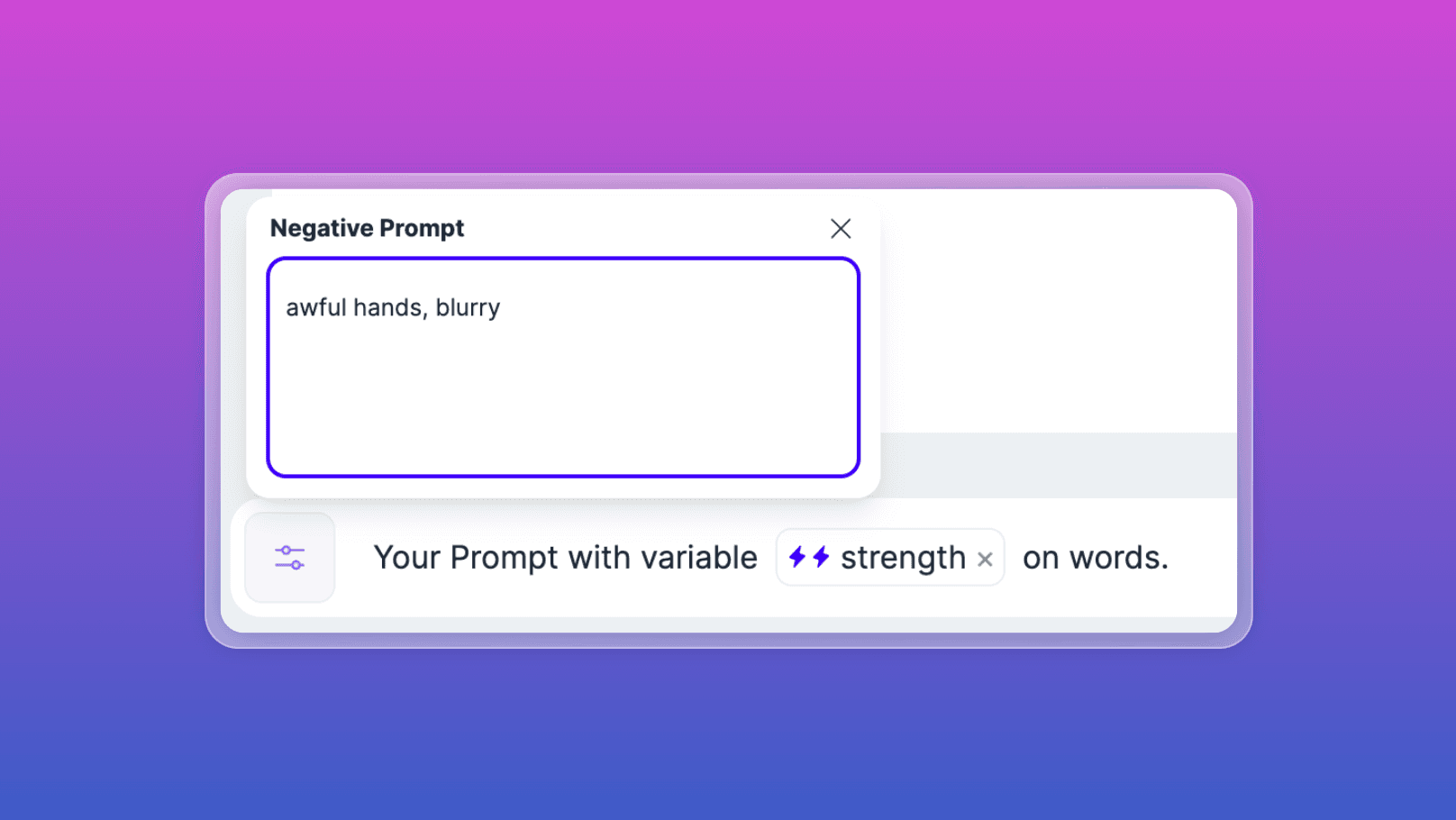
Usage
To use the PromptInput component, you need to manage its state from a parent component, handling both the weighted prompts and the negative prompt.
page.tsx
1
2 import React, { useState } from 'react';
3 import PromptInput from './PromptInput';
4
5 export const PromptInputDemo = () => {
6 const [prompts, setPrompts] = useState([]);
7 const [negativePrompt, setNegativePrompt] = useState("");
8
9 return (
10 <div className="...">
11 <PromptInput
12 negativePrompt={negativePrompt}
13 setNegativePrompt={setNegativePrompt}
14 prompts={prompts}
15 setPrompts={setPrompts}
16 onClick={() => alert("generate clicked")}
17 />
18 </div>
19 );
20 };
21
Weighted Prompts Format
The prompts state should be an array of objects, each representing a part of the prompt with an associated importance level.
prompts.ts
1
2 [
3 {"text": "text part", "importance": importance level},
4 ...
5 ]
Importance Levels:
- -> 0: Normal importance.
- -> 1: Medium importance.
- -> 2: High importance.
Converting it to weighted prompt
Most of the generative image AI models use a weighted prompt syntax for the input.Ex: penguin (holding:1.1) (a beer:1.3)
You can implement a utility function to achieve desired output like following:
prompt-utils.ts
1
2 function convertWeightedPromptsToString(prompts) {
3 // Map each prompt to a string, adding the importance directly without modification
4 const promptStrings = prompts.map(prompt => {
5 if (prompt.importance === 0) {
6 return prompt.text; // No importance shown for 0
7 }
8 // Adjusting the importance format as per your requirement
9 const adjustedImportance = prompt.importance === 1 ? "1.1" : "1.3";
10 return `(${prompt.text}:${adjustedImportance})`;
11 });
12
13 // Join all prompt strings into one, separating them by spaces
14 return promptStrings.join(" ");
15 }
16
17 // Usage example
18 const weightedPrompts = [
19 { text: "penguin", importance: 0 },
20 { text: "holding", importance: 1 },
21 { text: "a beer", importance: 2 }
22 ];
23
24 const outputString = convertWeightedPromptsToString(weightedPrompts);
25 console.log(outputString);
26 // penguin (holding:1.1) (a beer:1.3)